Implementing a MQTT Publisher-Subscriber Model with Support for Multiple Publishers
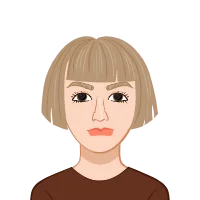
As the world becomes more interconnected, the Internet of Things (IOT) has permeated various aspects of our daily lives, revolutionizing industries such as healthcare, agriculture, and transportation. IOT devices, ranging from smart thermostats to industrial sensors, play a pivotal role by incessantly gathering and relaying data. Amidst this data-driven revolution, MQTT (Message Queuing Telemetry Transport) emerges as a beacon of efficiency. Its lightweight and robust publish-subscribe messaging protocol have made MQTT the go-to choice for IOT applications, facilitating seamless communication between devices and systems, while conserving precious bandwidth and energy resources.
In today's interconnected world, where the Internet of Things is rapidly shaping industries, mastering MQTT is more critical than ever, especially if you're seeking assistance with your IOT assignment using MQTT. MQTT's ability to handle multiple publishers efficiently ensures that IOT ecosystems can scale seamlessly. In this blog post, we will explore how to implement an MQTT publisher-subscriber model with support for multiple publishers, making it a valuable resource for students studying IOT and needing assistance with their assignments. This knowledge will enable them to grasp the fundamental concepts of MQTT and learn how to build scalable IoT applications, ultimately empowering them to contribute to the exciting evolution of IOT technology.
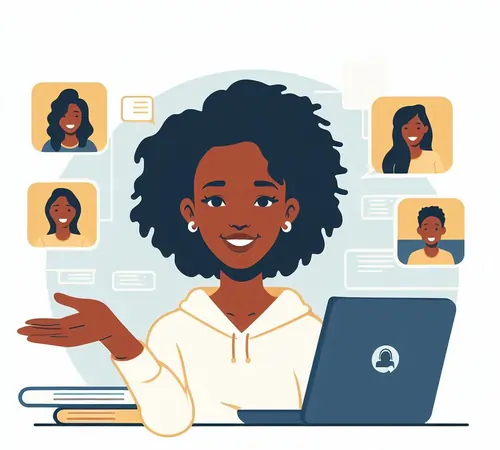
What is MQTT?
Before we dive into the implementation details, let's briefly discuss what MQTT is and why it's so important in the world of IOT.MQTT, or Message Queuing Telemetry Transport, serves as the backbone of many IOT ecosystems due to its lightweight nature and efficiency. Unlike traditional request-response protocols, MQTT operates on a publish-subscribe model, reducing constant polling and conserving both bandwidth and power. This design is particularly crucial for resource-constrained devices, common in IOT applications, as it minimizes the energy required for data transmission.
Furthermore, MQTT's support for Quality of Service (QoS) levels ensures that data can be delivered reliably, making it suitable for applications where data integrity is paramount, such as remote monitoring, industrial automation, and smart homes. Its ability to handle multiple publishers and subscribers seamlessly facilitates the scalability of IOT systems, allowing them to grow and adapt to changing requirements. Understanding MQTT is, therefore, pivotal for any student aiming to become proficient in IOT development.
MQTT Basics
MQTT's lightweight and efficient nature, coupled with its support for low-bandwidth, high-latency, and unreliable networks, positions it as the protocol of choice for IOT applications. Its origins in IBM's late-1990s development showcase its enduring legacy as a reliable and adaptable solution for facilitating seamless communication between a vast array of IOT devices and applications. This protocol's ability to gracefully handle the challenges posed by the diverse IOT landscape has solidified MQTT's status as an industry-standard, underpinning the backbone of countless smart systems and solutions worldwide.
Key Concepts in MQTT
Certainly, let's delve deeper into the key concepts of MQTT (Message Queuing Telemetry Transport) to gain a comprehensive understanding of this lightweight and efficient messaging protocol. Here are some given below:
- Publishers: Devices that produce data and send it to the MQTT broker. Data is organized into topics. Publishers play a critical role in the MQTT ecosystem by generating and dispatching data messages to specific topics, which act as channels for data distribution. These devices can range from environmental sensors collecting temperature data to industrial machines reporting operational status.
- Subscribers: Devices or applications that subscribe to specific topics to receive data updates from publishers. Subscribers are the recipients of data in an MQTT network. They express interest in particular topics and receive relevant data updates from publishers. Subscribers can be anything from a smartphone app displaying real-time weather information to a control system reacting to changes in sensor readings.
- Broker: The MQTT server that acts as an intermediary between publishers and subscribers. It receives published messages and forwards them to interested subscribers. The MQTT broker is the central hub that manages message routing and ensures efficient communication between publishers and subscribers. It plays a vital role in decoupling publishers and subscribers, allowing them to operate independently.
- Topics: Hierarchical identifiers that categorize published data. Subscribers can subscribe to specific topics to receive relevant data. Topics provide a structured way to organize data within the MQTT ecosystem. They are like channels or categories that help subscribers filter and receive only the information they are interested in. For instance, in a home automation system, topics could be used to differentiate between lighting control, thermostat settings, and security alerts.
- QoS (Quality of Service): MQTT supports three levels of QoS, which determine the message delivery guarantees. QoS 0 (At most once), QoS 1 (At least once), and QoS 2 (Exactly once). These QoS levels enable users to define the reliability and delivery assurance of messages. For example, QoS 0 is suitable for non-critical data where some loss is acceptable, while QoS 2 ensures that messages are delivered exactly once, even in the face of network disruptions. Understanding and selecting the appropriate QoS level is essential when designing MQTT applications.
Now that we have a basic understanding of MQTT, let's move on to the implementation.
Setting Up the Environment
To implement an MQTT publisher-subscriber model with support for multiple publishers, we need to ensure that our MQTT broker is capable of handling concurrent connections and message distribution efficiently. Additionally, it's essential to maintain a clear topic hierarchy that allows publishers and subscribers to easily identify and share relevant data streams. Proper security measures, such as authentication and encryption, should also be in place to safeguard the integrity of the MQTT communication. In this comprehensive guide, we'll delve into these crucial aspects while providing a step-by-step approach to building a robust multi-publisher MQTT system, we need a few components:
- MQTT Broker: You can use open-source MQTT brokers like Mosquitto, Eclipse Mosquitto, or HiveMQ. These brokers will handle the communication between publishers and subscribers. Mosquitto, for instance, is known for its lightweight nature and wide community support, making it an excellent choice for small to medium-scale IOT projects.
- Programming Language: Choose a programming language for your implementation. MQTT libraries are available for a wide range of languages, including Python, Java, JavaScript, and C/C++. Python is often favored for its simplicity and extensive libraries, making it a great choice for beginners in IOT development.
- MQTT Client Libraries: Depending on the programming language you choose, you'll need to install the appropriate MQTT client library. For example, for Python, you can use the popular Paho MQTT library. Paho MQTT offers comprehensive documentation and active development, ensuring reliability and ease of use in your Python-based IOT projects.
Implementing the MQTT Publisher
Let's start by implementing an MQTT publisher. In this example, we'll use Python and the Paho MQTT library, a widely adopted choice for MQTT communication in Python due to its robust features and community support. With Paho MQTT, you can easily create MQTT clients, handle connections, and publish data to MQTT topics, making it an excellent tool for building IOT applications and experimenting with the MQTT protocol.
Step 1: Install the Paho MQTT Library
You can install the Paho MQTT library using pip, a popular package manager for Python. Open your terminal or command prompt and run the following command to install the library:
pip install paho-mqtt
Ensure that you have Python and pip installed on your system before executing this command. Once installed, you can start building MQTT publishers and subscribers in Python effortlessly.
Step 2: Write the Publisher Code
Here's a simple Python script that publishes messages to an MQTT topic:
import paho.mqtt.client as mqtt
# Define the MQTT broker address and port
broker_address = "mqtt.eclipse.org"
port = 1883
# Create an MQTT client
client = mqtt.Client("Publisher")
# Connect to the broker
client.connect(broker_address, port)
# Publish a message to a topic
topic = "iot/sensor/data"
message = "Hello, MQTT!"
client.publish(topic, message)
# Disconnect from the broker
client.disconnect()
This code establishes a connection to an MQTT broker, publishes a message to the "iot/sensor/data" topic, and then disconnects from the broker.
Implementing Multiple Publishers
To support multiple publishers, you can create additional instances of the publisher script, each with a unique client ID. This allows you to have multiple devices or applications publishing data simultaneously. Each publisher can choose to publish messages to its designated topic, ensuring organized data distribution. Moreover, publishers can also share topics with other publishers if collaborative data sharing is required. This flexibility enables the construction of complex IOT ecosystems where various devices, sensors, or software components can seamlessly contribute data to the MQTT network, fostering efficient communication and data exchange among them.
Implementing the MQTT Subscriber
Now, let's implement an MQTT subscriber that can receive messages from multiple publishers. MQTT subscribers are essential components in an IOT ecosystem, as they enable devices and applications to stay updated with real-time data from various sources.
In the provided Python script, we have defined a callback function, on_message, which gets triggered whenever a message is received on the subscribed topic. This callback can be customized to perform specific actions based on the received data, making it a versatile tool for processing incoming MQTT messages.
By deploying multiple instances of this subscriber script with different client IDs and subscribed topics, you can efficiently collect data from numerous publishers and integrate it into your IOT applications. This scalability and flexibility are crucial for building robust and responsive IOT solutions.
Step 1: Write the Subscriber Code
Here's a Python script that subscribes to an MQTT topic and receives messages:
import paho.mqtt.client as mqtt
# Define the MQTT broker address and port
broker_address = "mqtt.eclipse.org"
port = 1883
# Callback when a message is received
def on_message(client, userdata, message):
print(f"Received message '{message.payload.decode()}' on topic '{message.topic}'")
# Create an MQTT client
client = mqtt.Client("Subscriber")
# Set the message callback
client.on_message = on_message
# Connect to the broker
client.connect(broker_address, port)
# Subscribe to a topic
topic = "iot/sensor/data"
client.subscribe(topic)
# Start the MQTT loop to receive messages
client.loop_forever()
This code establishes a connection to the MQTT broker, subscribes to the "iot/sensor/data" topic, and prints received messages.
Supporting Multiple Subscribers
To support multiple subscribers, you can create additional instances of the subscriber script, each with a unique client ID. This approach allows you to distribute the workload among multiple subscribers, making your MQTT-based system more scalable and responsive. Each subscriber can either subscribe to its designated topic, enabling specialized data processing, or share common topics with other subscribers for broader data access. This flexibility ensures that you can tailor your MQTT subscriber architecture to meet the specific requirements of your IOT application, whether it involves data aggregation, real-time monitoring, or data analysis. By designing your MQTT subscribers effectively, you can harness the full potential of MQTT in managing IOT data streams.
Conclusion
Implementing an MQTT publisher-subscriber model with support for multiple publishers is a fundamental skill for students interested in IOT. MQTT is a robust and efficient protocol that enables communication between IOT devices and applications, making it a crucial technology in the IOT ecosystem.
In this blog post, we've covered the basics of MQTT, set up the development environment, and provided code examples for implementing MQTT publishers and subscribers. Remember that there are various MQTT brokers and client libraries available in different programming languages, allowing you to choose the tools that best suit your project's requirements.
As students, exploring MQTT and building your own IOT applications will not only enhance your understanding of IOT principles but also prepare you for real-world IOT development tasks. So, go ahead, experiment with MQTT, create your own MQTT-based projects, and dive deeper into the fascinating world of the Internet of Things.