Simulating Network Routers Using C for Network Programming

In the realm of computer networking, understanding the intricacies of network routers is essential for any aspiring network engineer or computer science student. Routers are the backbone of the internet, responsible for directing data packets to their destinations. To gain a deeper understanding of how routers work, students often encounter assignments that involve simulating network routers. If you're looking for guidance on how to write your network programming Assignment, in this blog post, we will explore the concept of simulating network routers using the C programming language for network programming purposes. We'll cover the fundamentals, offer guidance for tackling assignments, and provide some helpful code snippets.
Understanding the Basics of Network Routers
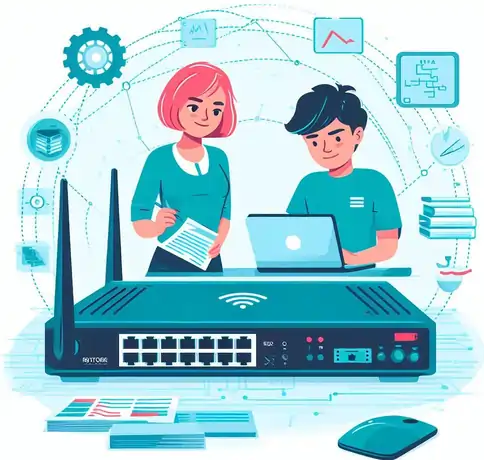
Before diving into the world of network router simulation, let's establish a solid understanding of what a network router is and its role in the networking landscape. This foundational knowledge is essential as it forms the bedrock upon which the entire simulation process is built. Just as an architect must comprehend the intricacies of building materials and design principles, a network engineer or programmer embarking on router simulation must grasp the fundamental concepts that underpin the operation of these critical devices. With this knowledge as our compass, we can embark on our journey to delve into the fascinating world of network router simulation using the C programming language.
What Is a Network Router?
A network router is a device that connects different computer networks together and directs data packets between them. It operates at the network layer (Layer 3) of the OSI model and makes decisions based on routing tables to determine the most efficient path for data to travel. Routers play a crucial role in ensuring that data packets reach their intended destinations, whether those destinations are within a local network or on the global internet.
A network router serves as the traffic cop of the digital world, ensuring seamless communication across diverse networks. As a pivotal component of the OSI model's Layer 3, it not only handles routing tables but also actively manages congestion and adapts to network changes in real time. In a constantly evolving digital landscape, routers remain at the forefront of maintaining efficient data flow, making them indispensable for the internet's continued operation.
Key Functions of a Router
Routers are pivotal devices in the world of networking, performing a multitude of functions to ensure efficient data transmission between networks. Here, we delve deeper into the primary functions of a router:
Packet Forwarding: Routers are responsible for forwarding data packets between networks. They examine the destination IP address of each packet and use routing tables to determine the next hop. Packet forwarding is a critical function of routers that ensures seamless data transmission between networks. Routers inspect the destination IP address of each incoming packet, enabling them to determine the optimal path for data traversal. This intelligent routing process is fundamental to the efficient operation of the internet.
Routing Tables: Routing tables contain information about network topology and available routes. They help routers make informed decisions about where to send data packets. It serve as the backbone of a router's decision-making process. They contain essential information about network topology and available routes, offering a roadmap for routers to follow. These tables are dynamic, allowing routers to adapt to changing network conditions and make informed decisions about where to route data packets.
Network Address Translation (NAT): Routers can perform NAT, which allows multiple devices within a local network to share a single public IP address. Network Address Translation (NAT) is a router feature with transformative implications for local networks. NAT allows multiple devices within a local network to share a single public IP address. By mapping internal IP addresses to the router's public IP, NAT enables a household or organization to efficiently utilize a limited pool of public addresses, thus extending the life of IPv4.
Firewall: Routers often include firewall capabilities to filter incoming and outgoing traffic, enhancing network security. Incorporating firewall capabilities, routers bolster network security by acting as gatekeepers. They scrutinize incoming and outgoing traffic, identifying potential threats or unauthorized access attempts. Firewalls implement rule-based policies that filter data packets, safeguarding networks from malicious actors and ensuring data integrity.
Quality of Service (QoS): Some routers can prioritize certain types of traffic, ensuring a better user experience for applications like VoIP or video streaming. Quality of Service (QoS) is a feature that elevates routers to sophisticated traffic managers. Some routers can prioritize certain types of traffic based on defined criteria. This ensures a superior user experience for applications such as VoIP or video streaming, where latency and packet loss can be particularly detrimental. By allocating network resources wisely, QoS enhances overall network performance.
Simulating Network Routers with C
Simulating network routers using the C programming language is a challenging yet rewarding task. It allows students to gain hands-on experience and a deeper understanding of how routers process and forward data packets. Below are the key steps involved in creating a basic router simulation in C:
Step 1: Data Structures
Start by defining data structures to represent the router's components, such as routing tables, data packets, and network interfaces. These data structures will serve as the building blocks of your simulation, allowing you to store and manipulate information about routes, packet headers, and the router's connection points. Ensure that these structures accurately mirror the real-world counterparts to maintain fidelity in your simulation. Additionally, consider incorporating dynamic data structures like linked lists or hash tables to efficiently manage routing entries as your simulation evolves. Here's a simplified example:
struct Packet {
char source_ip[15];
char dest_ip[15];
char payload[1024];
};
struct RoutingTableEntry {
char network[15];
char next_hop[15];
char interface[10];
};
Step 2: Routing Table Initialization
Creating a function to initialize the routing table with sample entries is a crucial step in router simulation. Begin by defining your routing table structure and allocating memory for it. Populate the table with network addresses, next-hop IP addresses, and associated interfaces. This initialization will serve as the foundation for routing decisions in your simulation. Ensure that you include both static and dynamic routing entries to mimic real-world scenarios.
Step 3: Packet Forwarding Logic
The implementation of packet forwarding logic is the heart of your router simulation. Upon receiving a packet, your router should examine the destination IP address using bitwise operations to match it with entries in the routing table. To forward the packet accurately, consider incorporating algorithms like Longest Prefix Match (LPM) to determine the best route. Additionally, logging the packet's journey through the router can be valuable for debugging and analysis purposes. Ensure that your forwarding logic efficiently utilizes the routing table for optimal network performance. Here's a simplified example:
void forwardPacket(struct Packet packet) {
// Search the routing table for the best route
// Update the packet's destination and interface
// Forward the packet to the next hop
}
Step 4: Network Interface Handling
Simulate network interfaces as sockets or ports. Create functions to send and receive packets through these interfaces. You can use socket programming in C to achieve this. To manage network interfaces effectively, consider using separate threads for each interface to handle concurrent communication. Ensure proper error handling for socket operations to maintain the robustness of your simulation.
Step 5: Main Loop
In your main program loop, continuously check for incoming packets on network interfaces and invoke the packet forwarding logic. Implement a timer mechanism to periodically update routing tables if your simulation includes dynamic routing protocols like OSPF or RIP. This ensures that your router adapts to network changes in real-time.
Step 6: Testing and Debugging
Test your router simulation with sample data packets and routing scenarios. Implement debugging mechanisms to trace the packet's journey through the router. Utilize packet capture tools like Wireshark to inspect packets at various stages of processing within your simulated router. This visual debugging approach can be invaluable in identifying and resolving issues.
Step 7: Dynamic Routing (Optional)
If your assignment involves dynamic routing protocols, extend your simulation by implementing algorithms like Dijkstra's or Bellman-Ford to calculate optimal routes dynamically. Monitor the protocol-specific messages exchanged between routers to ensure correct operation, and implement mechanisms to handle route updates and convergence.
Step 8: Advanced Features (Optional)
Consider adding advanced features such as Network Address Translation (NAT), firewall rules, and Quality of Service (QoS) policies to make your router simulation more comprehensive. This will provide you with a deeper understanding of the multifaceted roles that modern routers play in network environments.
Tips for Students Working on Router Simulation Assignments
Creating a network router simulation can be complex, but it's an excellent opportunity for students to enhance their understanding of networking concepts and programming skills. Here are some tips to help students excel in their router simulation assignments:
Start Simple: Begin with a basic router simulation and gradually add complexity as you gain confidence. Start by forwarding packets based on a static routing table before implementing dynamic routing protocols. This step-by-step approach allows you to build a strong foundation and grasp fundamental routing concepts before tackling more advanced features like OSPF or BGP.
Use Libraries: Leverage networking libraries like libpcap or WinPcap for packet capture and analysis. These libraries can simplify the handling of network interfaces and packets, saving you valuable time and effort in low-level network programming. They provide pre-built functions for tasks like packet sniffing and inspection.
Documentation and Comments: Document your code thoroughly, including comments that explain the purpose of functions and data structures. Clear documentation will make it easier to troubleshoot and debug, as well as help future developers understand your code. Well-commented code is not just a best practice; it's a lifesaver during complex projects.
Modular Design: Divide your code into separate modules or functions, each responsible for a specific task. This promotes code reusability and maintainability. By breaking your router simulation into manageable components like packet handling, routing table management, and interface handling, you can easily modify and enhance individual parts without affecting the entire system.
Error Handling: Implement robust error handling to gracefully handle unexpected situations, such as network interface failures or invalid packet formats. Proper error handling prevents your simulation from crashing and provides valuable insights into potential issues during development. It also demonstrates your ability to create a reliable and resilient system.
Research and Reference: Consult textbooks, online resources, and reference books on computer networking and C programming. Understanding the theory behind routing algorithms and protocols is crucial. Stay up-to-date with the latest networking standards and practices to ensure your simulation aligns with real-world scenarios and requirements.
Peer Collaboration: Collaborate with peers and seek help from professors or mentors when facing challenges. Group discussions and peer reviews can provide valuable insights. Sharing different perspectives can lead to innovative solutions, and learning from each other's mistakes can help you avoid common pitfalls in router simulation development. Building a network of fellow students interested in the subject can be beneficial for future endeavors as well.
Project Presentation: When presenting your project, explain the design decisions you made, the algorithms you used, and the challenges you encountered. A well-structured presentation can impress your instructors. Additionally, consider demonstrating the practical applications and real-world relevance of your router simulation. Highlight how your project contributes to a deeper understanding of network routing, making it easier for your audience to appreciate the significance of your work.
Feedback and Iteration: While collaborating with peers and presenting your project, be open to feedback and constructive criticism. Peer input can help you identify blind spots in your simulation, refine your code, and enhance your overall project quality. Use feedback as an opportunity for iteration, continually improving your router simulation to meet higher standards.
Community Engagement: Extend your peer collaboration beyond your immediate circle. Engage with online communities, forums, or social media groups related to networking and programming. Sharing your experiences and challenges can lead to valuable insights from a global network of enthusiasts and professionals who have tackled similar projects.
Documentation Excellence: In your project presentation, emphasize the importance of clear and comprehensive documentation. Proper documentation not only helps you understand and maintain your code but also makes it accessible to others who may want to explore or build upon your work. Consider creating user manuals or README files to guide users and contributors through your router simulation project.
Conclusion
Simulating network routers using C for network programming assignments is an educational journey that can significantly enhance your knowledge of computer networking. Understanding the basics of network routers, following a structured development approach, and adhering to best practices in coding will set you on the path to success in completing your assignments. Embrace the challenges, stay curious, and enjoy the learning process!
Additionally, remember that network router simulation is not only an academic exercise but also a valuable skill in the real world of networking. As you progress in your studies and career, the hands-on experience gained from these assignments will prove invaluable. Networking is a dynamic field, and the ability to comprehend and simulate routers will enable you to adapt to evolving technologies and make meaningful contributions to the ever-expanding world of network engineering. So, dive into the world of router simulation with enthusiasm, and you'll discover a profound appreciation for the backbone of modern communication systems.